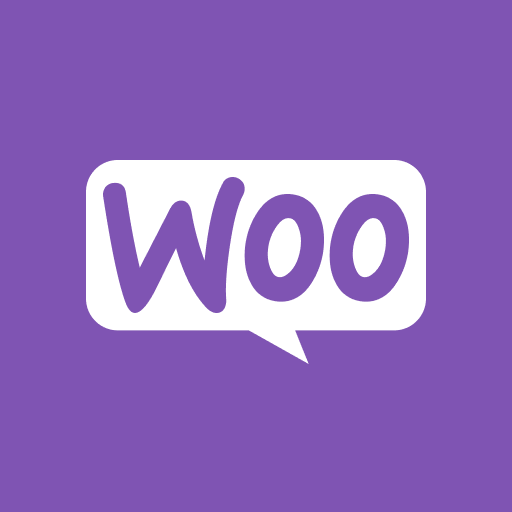
WooCommerce REST API is useful for creating headless shops and automating processes . Here we will show couple of examples where you can see how our plugins can be managed via Woo REST api like how to retrieve product data and how to create new auction product using WooCommerce REST API v3.
First you will need to go to WooCommerce Settings -> Advanced and enable REST API then create client key and secret, then assign permissions you need for your use case.
Once this is completed go to https://github.com/Automattic/wp-rest-php-lib and download their PHP lib. Extract so you have folder structrue something like https://www.yourwebsite.com\api\WooCommerce and in \api\ create test_api.php file. Their lib is intented to be used with Composer and we advise that but in the example we will not use Composer in order to have as less prerequisites as possible.
Code example for WooCommerce REST API – listing auctions and creating new auction product
Since WooCommerce REST API can retrieve custom product types we will use type parameter to retrieve all auction products available in our Woo product list. Copy paste into your test_api.php file following code:
<?php
include_once(__DIR__ . '/WooCommerce/HttpClient/BasicAuth.php');
include_once(__DIR__ . '/WooCommerce/HttpClient/HttpClient.php');
include_once(__DIR__ . '/WooCommerce/HttpClient/HttpClientException.php');
include_once(__DIR__ . '/WooCommerce/HttpClient/OAuth.php');
include_once(__DIR__ . '/WooCommerce/HttpClient/Options.php');
include_once(__DIR__ . '/WooCommerce/HttpClient/Request.php');
include_once(__DIR__ . '/WooCommerce/HttpClient/Response.php');
include_once(__DIR__ . '/WooCommerce/Client.php');
use Automattic\WooCommerce\Client;
$woocommerce = new Client(
'https://www.yourwebsite.com',
'your_client_key',
'your_client_secret',
[
'wp_api' => true,
'version' => 'wc/v3',
]
);
$data = [
'type' => 'auction' // get all auction products
];
print_r( $woocommerce->get( 'products', $data) );
Examples how to get and create auction products (for WooCommerce Simple Auctions) using WooCommerce API:
$data = [
'name' => 'New Auction',
'type' => 'auction',
'status' => 'draft', // status can be: draft, published, pending
'meta_data' => array(
[ 'key' => '_auction_start_price', 'value' => '7.99' ], // starting bid
[ 'key' => '_auction_bid_increment', 'value' => '1.5' ], // min bid increment
[ 'key' => '_regular_price', 'value' => '2900' ], // buy now price
[ 'key' => '_auction_reserved_price', 'value' => '2000.0' ], // reserve price
[ 'key' => '_auction_dates_from', 'value' => '2022-02-11 00:00' ], // start date
[ 'key' => '_auction_dates_to', 'value' => '2022-02-27 00:00' ], // end date
[ 'key' => '_auction_proxy', 'value' => 'no' ], // proxy auction, yes | no
[ 'key' => '_auction_sealed', 'value' => 'no' ], // sealed auction, yes | no
),
'description' => 'This is sample auction added via WooCommerce API',
'short_description' => 'Sample api auction.',
];
print_r( $woocommerce->post( 'products', $data ) );
Examples how to get and create lottery products (for plugin WooCommerce Lottery) using WooCommerce API:
$data = [
'type' => 'lottery' // get all lottery products
];
print_r( $woocommerce->get( 'products', $data) );
$data = [
'name' => 'New Lottery',
'type' => 'lottery',
'status' => 'draft', // status can be: draft, published, pending
'meta_data' => array(
[ 'key' => '_min_tickets', 'value' => '30' ], // min number of tickets needed
[ 'key' => '_max_tickets', 'value' => '100' ], // max number of tickets
[ 'key' => '_max_tickets_per_user', 'value' => '100' ], // max per user (optional)
[ 'key' => '_lottery_num_winners', 'value' => '1' ], // number of winners
[ 'key' => '_lottery_price', 'value' => '20.99' ], // ticket price
[ 'key' => '_lottery_sale_price', 'value' => '15.99' ], // sale price (optional)
[ 'key' => '_lottery_dates_from', 'value' => '2022-02-11 00:00' ], // start date
[ 'key' => '_lottery_dates_to', 'value' => '2022-02-27 00:00' ], // end date
),
'description' => 'This is sample lottery added via WooCommerce API',
'short_description' => 'Sample api lottery.',
];
print_r( $woocommerce->post( 'products', $data ) );
Examples how to get and create group buy / deal products (for plugin WooCommerce Lottery) using WooCommerce API:
$data = [
'type' => 'groupbuy' // get all group buy / deal products
];
print_r( $woocommerce->get( 'products', $data) );
$data = [
'name' => 'New Group Buy',
'type' => 'groupbuy',
'status' => 'draft', // status can be: draft, published, pending
'meta_data' => array(
[ 'key' => '_groupbuy_min_deals', 'value' => '30' ], // min deals sold needed
[ 'key' => '_groupbuy_max_deals', 'value' => '100' ], // max deals sold
[ 'key' => '_groupbuy_max_deals_per_user', 'value' => '100' ], // max per user (optional)
[ 'key' => '_groupbuy_price', 'value' => '10.99' ], // deal price
[ 'key' => '_groupbuy_regular_price', 'value' => '21.99' ], // regular price
[ 'key' => '_groupbuy_dates_from', 'value' => '2022-02-11 00:00' ], // start date
[ 'key' => '_groupbuy_dates_to', 'value' => '2022-02-27 00:00' ], // end date
),
'description' => 'This is sample group buy added via WooCommerce API',
'short_description' => 'Sample api group buy.',
];
print_r( $woocommerce->post( 'products', $data ) );
How can you limit number of produtcs retrieved? You can use per_page attribute in $data like below (search, offset, order, order by etc options are available useful for paging and filtering) – all available parameters are listed here https://woocommerce.github.io/woocommerce-rest-api-docs/#list-all-products:
$data = [
'per_page' => 1, // api will return only 1 auction product
'type' => 'auction'
];
print_r( $woocommerce->get( 'products', $data) );
Complete REST API documentation can be found on https://woocommerce.github.io/woocommerce-rest-api-docs/. If you have additional questions on how WooCommerce REST API works with WooCommerce Simple Auctions, WooCommerce Lottery and WooCommerce Group Buy please open ticket here or contact us.